UITableViewの2つのスタイル「Plain」と「Groupd」の違いを説明します。
iOS 7より前のバージョンでは、Groupdでセルの囲みが丸くなるはっきした違いがありましたが、iOS 7以降では違いが分かりづらくなっています。
とはいえ違いが存在していることは確かなため、細かい違いを意識してスタイルを正しく使い分けることが重要となっています。
Plainスタイル
PlainとGroupdのテーブルビューの違いを確認するため、2つのセクションを持つテーブルビューの簡単なサンプルプログラムを作成します。
なおUITableViewをソースコードから生成する場合は、UITableView生成時にスタイルとして、UITableViewStylePlainまたはUITableViewStyleGroupedを指定できますが、今回はInterface Builderを使用する方法を解説します。
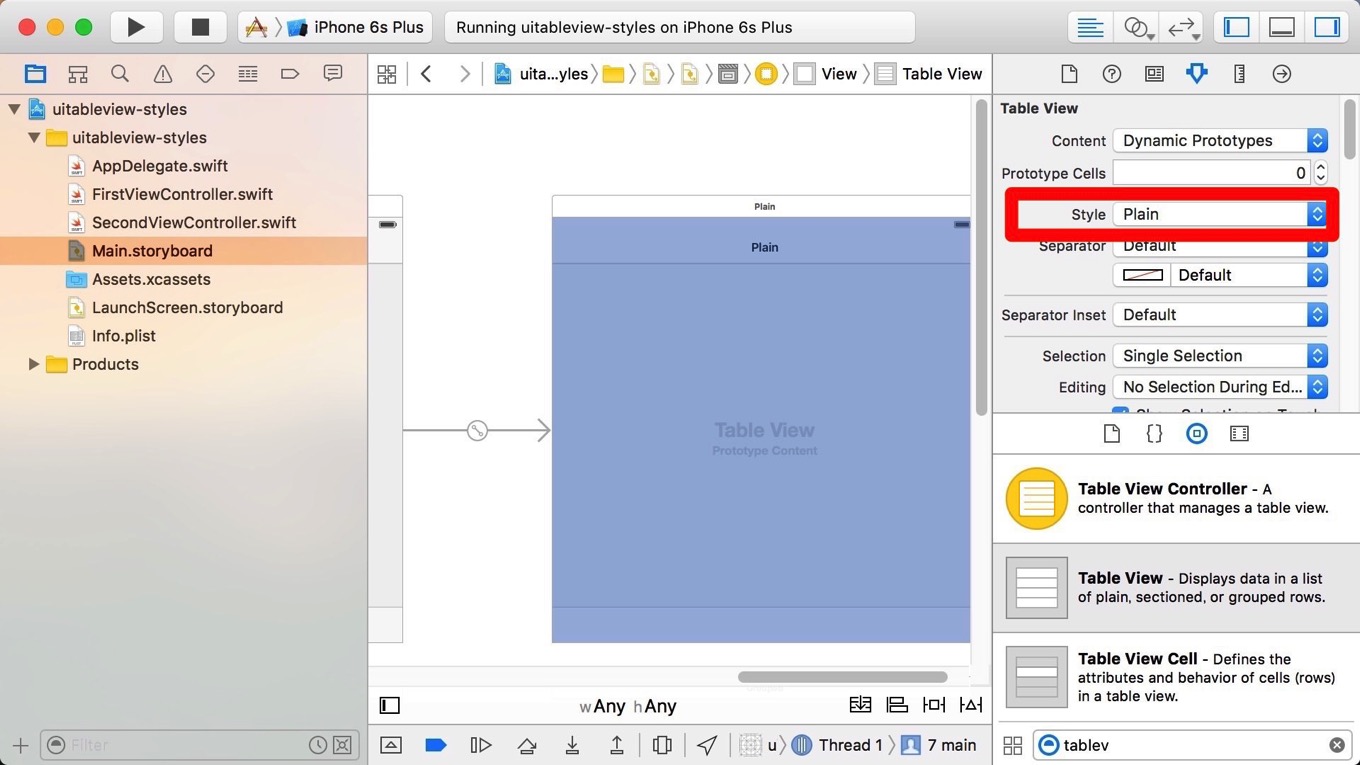
Main.storyboardをクリックし、Interface BuilderでView Controllerの上にTable Viewを設置します。Table ViewのスタイルをPlainに変更し、Table ViewのdataSourceをView Controllerに設定しておきます。
ソースコードを以下の様に実装します。
class FirstViewController: UIViewController, UITableViewDataSource {
@IBOutlet weak var tableView: UITableView!
var sections = ["国名", "都道府県"]
var countries = ["日本", "アメリカ", "中国", "ロシア", "ドイツ", "フランス"]
var prefectures = ["東京", "千葉", "神奈川", "埼玉", "大阪", "名古屋", "北海道"]
override func viewDidLoad() {
super.viewDidLoad()
tableView.registerClass(UITableViewCell.self, forCellReuseIdentifier: "myCell")
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
}
func numberOfSectionsInTableView(tableView: UITableView) -> Int {
return sections.count
}
func tableView(tableView: UITableView, titleForHeaderInSection section: Int) -> String? {
return sections[section]
}
func tableView(tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
var rows = 0
if section == 0 {
rows = countries.count
} else if section == 1 {
rows = prefectures.count
}
return rows
}
func tableView(tableView: UITableView, cellForRowAtIndexPath indexPath: NSIndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCellWithIdentifier("myCell", forIndexPath: indexPath)
var text = ""
var color = UIColor.whiteColor()
if indexPath.section == 0 {
text = countries[indexPath.row]
color = UIColor.grayColor()
} else if indexPath.section == 1 {
text = prefectures[indexPath.row]
color = UIColor.orangeColor()
}
cell.textLabel?.text = text
cell.backgroundColor = color
return cell
}
}
実行結果は以下の通り。
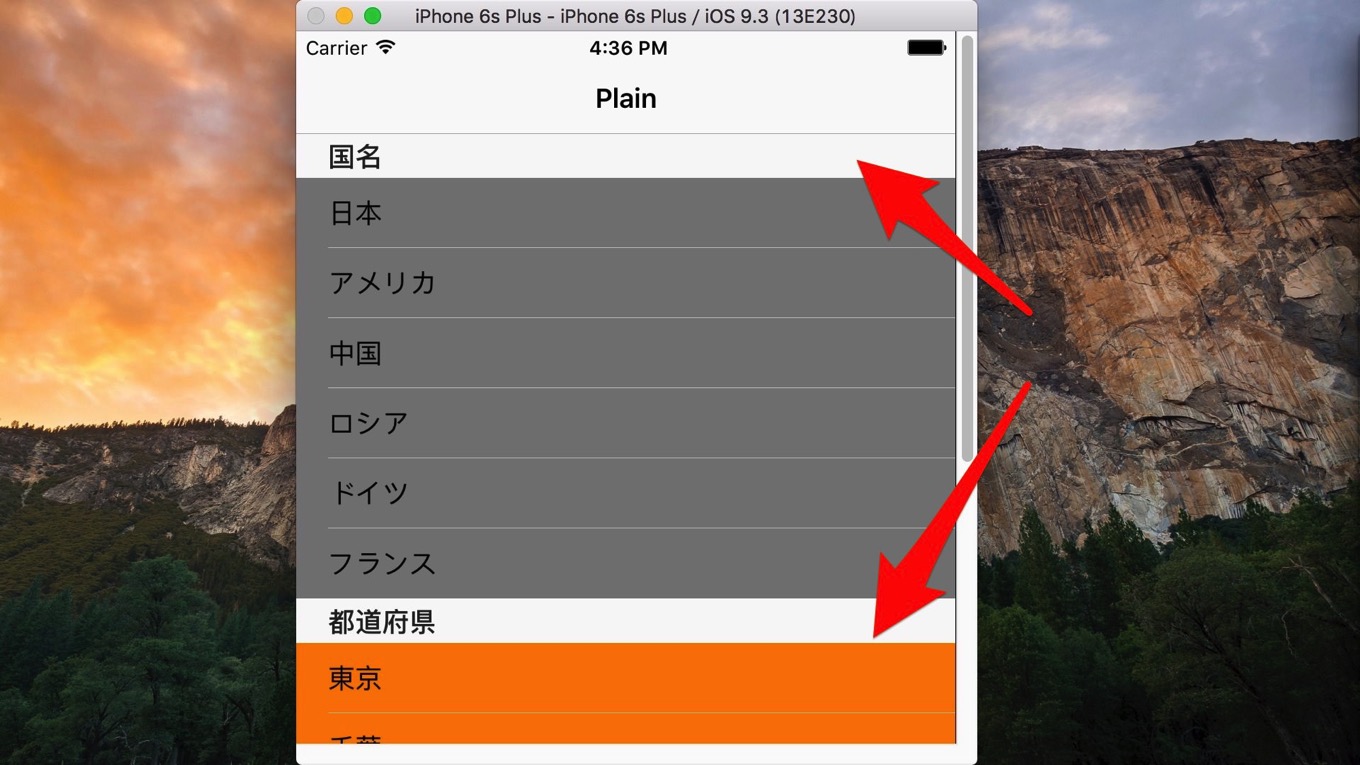
セクションヘッダーが1行分の高さで表示され、文字の色もはっきりとした黒い色で表示されています。スクロールした際セクションヘッダーが画面上部にフロート表示されるのも特徴です。
Groupedの場合
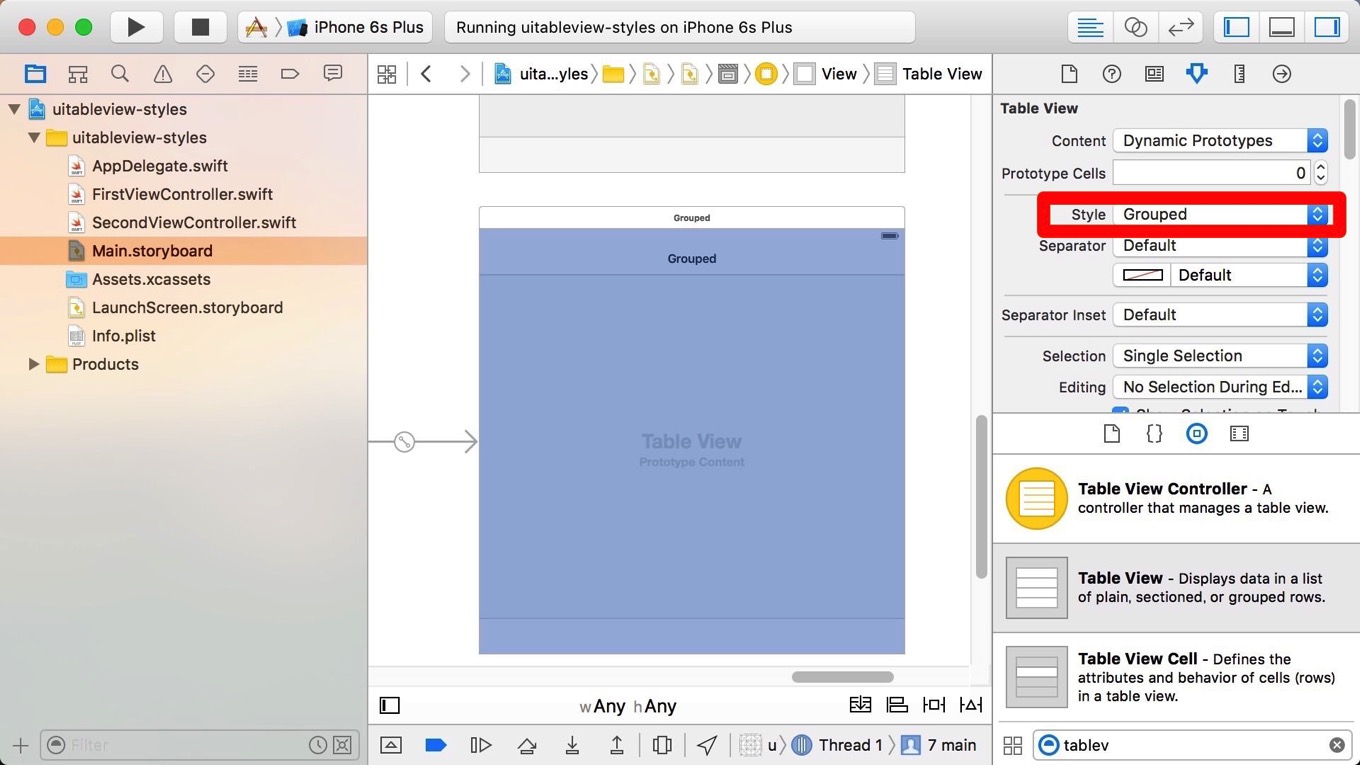
Groupedの場合のサンプルは、テーブルビューのStyleをGroupedに設定するところだけが異なります。ソースコードはPlainと全く同じです。
実行すると以下の様に表示されます。
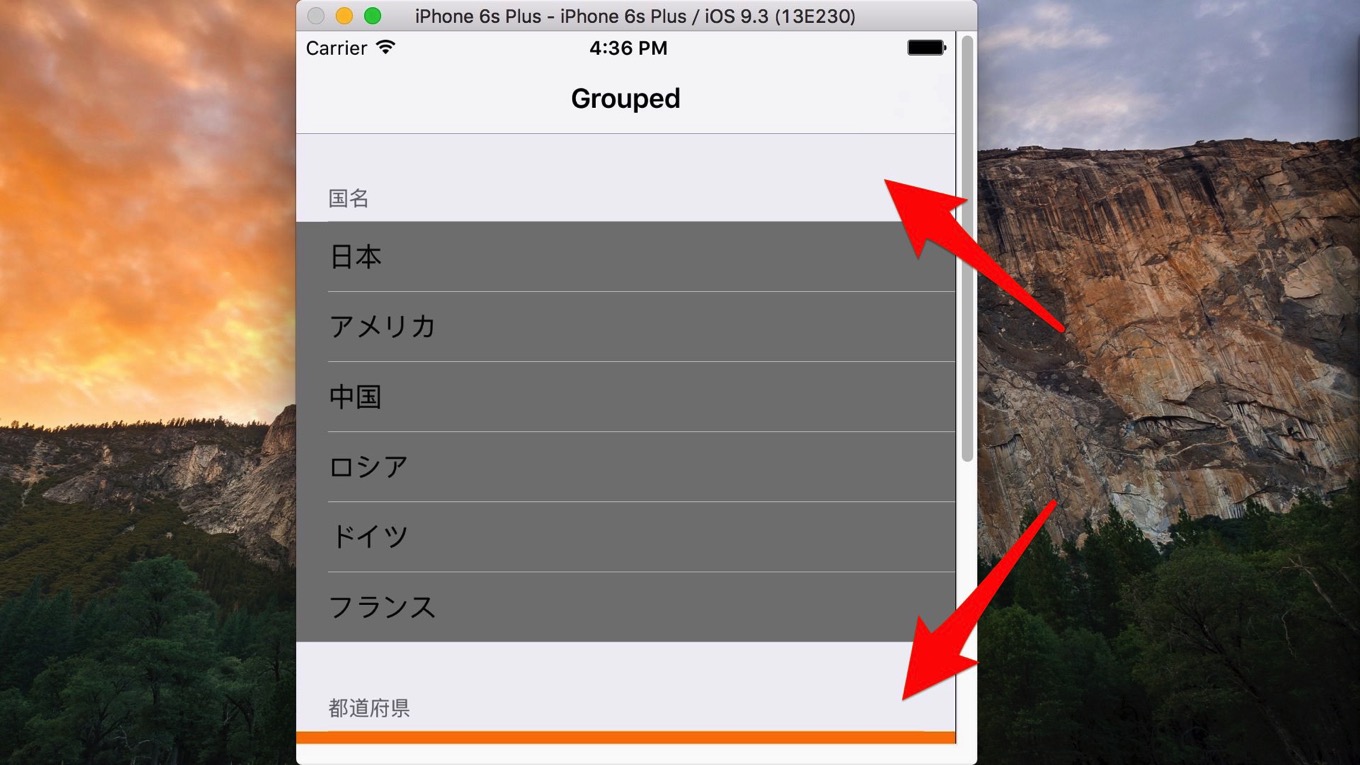
セクションヘッダーは2行分の高さで表示され、文字の色がグレーで表示されています。またフロート表示されずスクロールによって非表示となります。
まとめ
基本的にPlainは通常のデータの表示用に使用し、Groupedは設定値の編集用に使用するのが良さそうです。
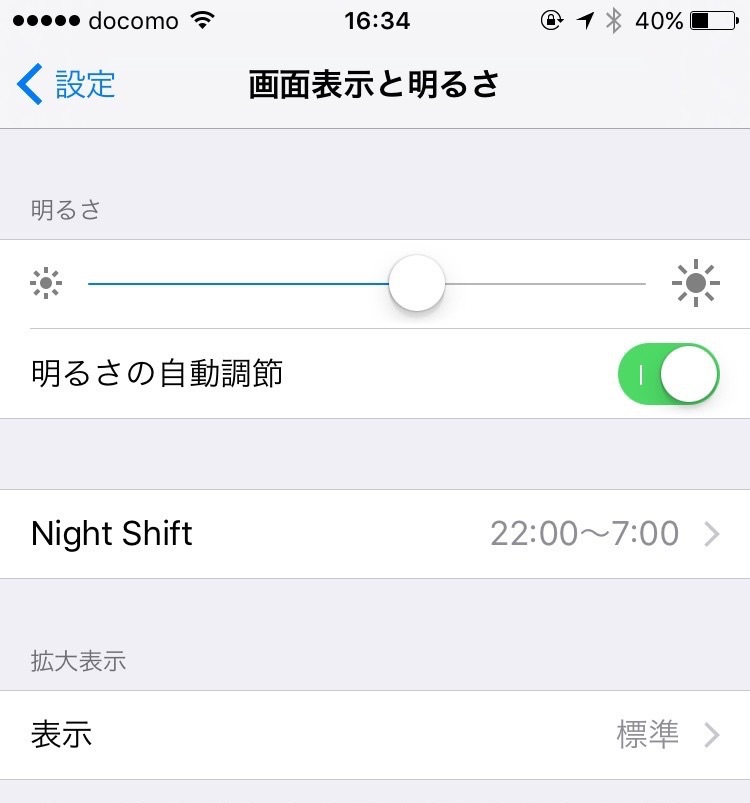
iOS標準の「設定アプリ」でもGroupdスタイルのテーブルビューが使用されていることが分かります。