画面にメッセージを表示したり、ユーザーに動作を選択してもらう際に使用するUIAlertControllerの基本的な使用方法を説明します。従来UIAlertViewやUIActionSheetを使っていたところですが、iOS 8以降これらのクラスの使用は推奨されていませんのでご注意ください。
基本
UIAlertControllerを生成し、アクションを追加(アクションのイベントハンドラはクロージャで処理)、presentViewControllerでUIAlertControllerを表示というのが基本的な流れです。
AlertとActionSheetはUIAlertControllerの引数で変更することができます。
let alert = UIAlertController(title: "アラート", message: "アラートのメッセージです", preferredStyle: UIAlertControllerStyle.Alert)
let cancelAction = UIAlertAction(title: "キャンセル", style: UIAlertActionStyle.Cancel, handler: {
(action:UIAlertAction!) -> Void in
print("キャンセル")
})
alert.addAction(cancelAction)
presentViewController(alert, animated: true, completion: nil)
Alertの表示
通常の処理を行うデフォルトボタン、アイテムの削除など破壊的な処理を行うDestructiveボタン、キャンセル処理を行うキャンセルボタンを追加したAlertを表示するサンプルです。
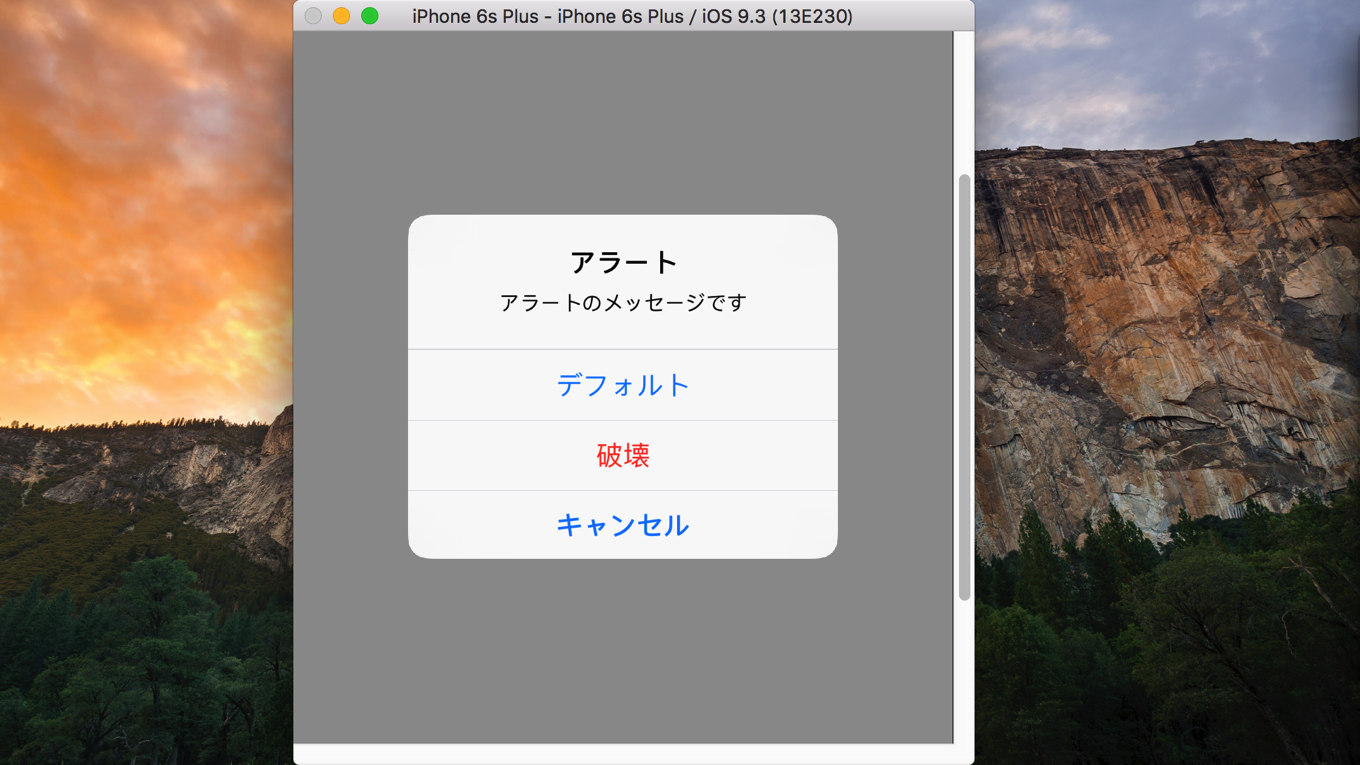
デフォルトボタン、Destructiveボタンは複数追加できるのに対し、キャンセルボタンは一つだけしか追加できないことに注意が必要です。
let alert = UIAlertController(title: "アラート", message: "アラートのメッセージです", preferredStyle: UIAlertControllerStyle.Alert)
//キャンセルアクション(1つだけ指定可能)
let cancelAction = UIAlertAction(title: "キャンセル", style: UIAlertActionStyle.Cancel, handler: {
(action:UIAlertAction!) -> Void in
print("キャンセル")
})
//デフォルトアクション(複数指定可能)
let defaultAction = UIAlertAction(title: "デフォルト", style: UIAlertActionStyle.Default, handler: {
(action:UIAlertAction!) -> Void in
print("デフォルト")
})
//破壊的アクション(複数指定可能)
let destructiveAction = UIAlertAction(title: "破壊", style: UIAlertActionStyle.Destructive, handler: {
(action:UIAlertAction!) -> Void in
print("破壊")
})
alert.addAction(cancelAction)
alert.addAction(defaultAction)
alert.addAction(destructiveAction)
presentViewController(alert, animated: true, completion: nil)
Action Sheetの表示
通常の処理を行うデフォルトボタン、アイテムの削除など破壊的な処理を行うDestructiveボタン、キャンセル処理を行うキャンセルボタンを追加したAction Sheetを表示するサンプルです。
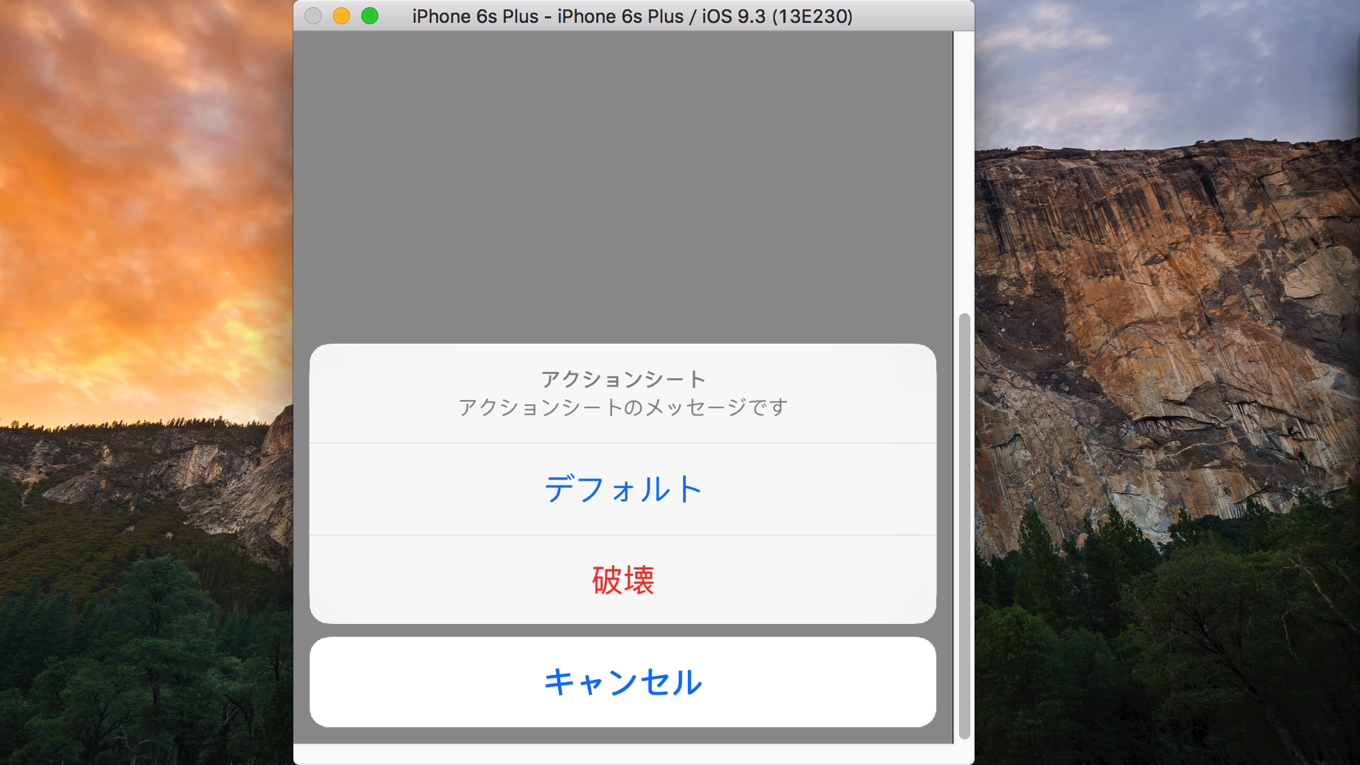
UIAlertControllerの引数が「UIAlertControllerStyle.ActionSheet」になっているだけで後はAlertを表示する場合と同じです。
let alert = UIAlertController(title: "アクションシート", message: "アクションシートのメッセージです", preferredStyle: UIAlertControllerStyle.ActionSheet)
//キャンセルアクション(1つだけ指定可能)
let cancelAction = UIAlertAction(title: "キャンセル", style: UIAlertActionStyle.Cancel, handler: {
(action:UIAlertAction!) -> Void in
print("キャンセル")
})
//デフォルトアクション(複数指定可能)
let defaultAction = UIAlertAction(title: "デフォルト", style: UIAlertActionStyle.Default, handler: {
(action:UIAlertAction!) -> Void in
print("デフォルト")
})
//破壊的アクション(複数指定可能)
let destructiveAction = UIAlertAction(title: "破壊", style: UIAlertActionStyle.Destructive, handler: {
(action:UIAlertAction!) -> Void in
print("破壊")
})
alert.addAction(cancelAction)
alert.addAction(defaultAction)
alert.addAction(destructiveAction)
presentViewController(alert, animated: true, completion: nil)
複雑なAction Sheetの表示
ボタンをさらに追加することもできます。
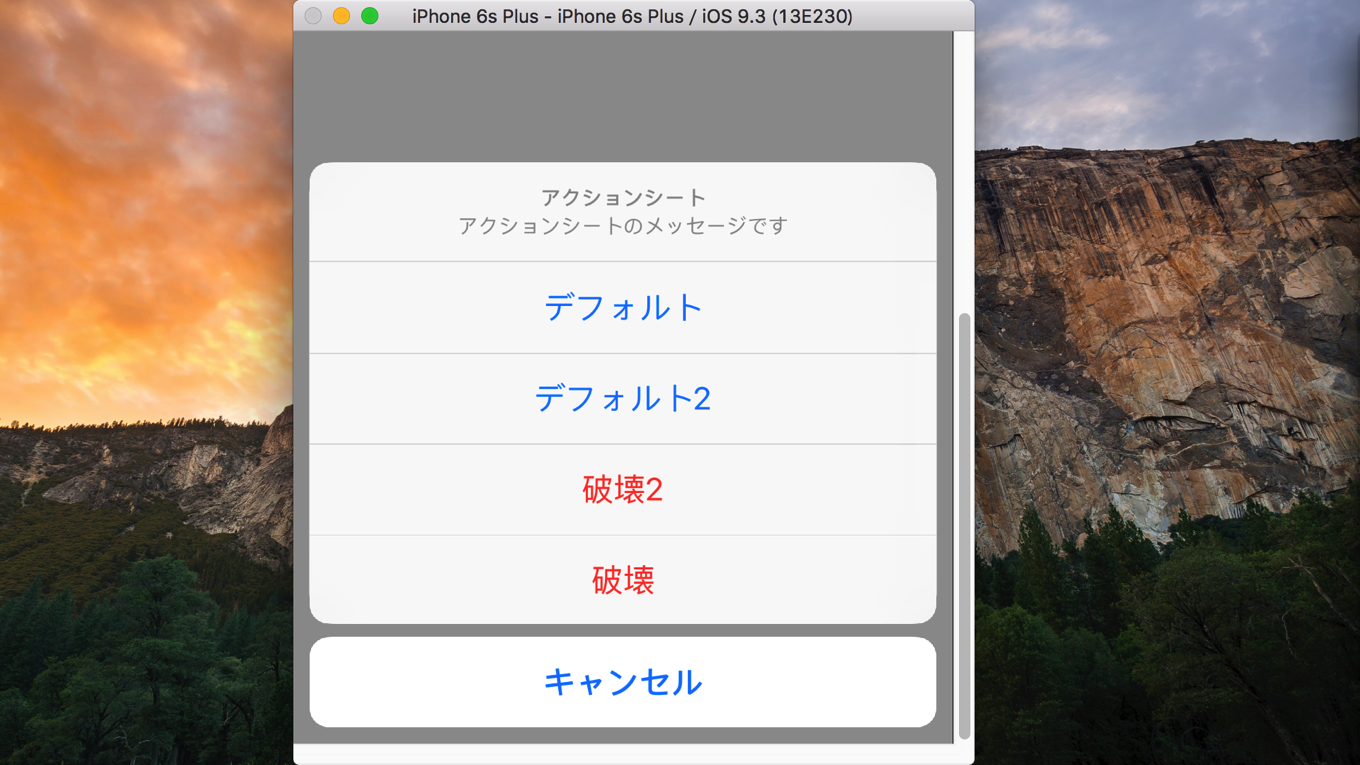
複数ボタンが追加できるデフォルトボタン、Destructiveボタンでは、addActionで追加した順番にボタンが表示されます。
let alert = UIAlertController(title: "アクションシート", message: "アクションシートのメッセージです", preferredStyle: UIAlertControllerStyle.ActionSheet)
//キャンセルアクション(1つだけ指定可能)
let cancelAction = UIAlertAction(title: "キャンセル", style: UIAlertActionStyle.Cancel, handler: {
(action:UIAlertAction!) -> Void in
print("キャンセル")
})
let cancel2Action = UIAlertAction(title: "キャンセル2", style: UIAlertActionStyle.Cancel, handler: {
(action:UIAlertAction!) -> Void in
print("キャンセル2")
})
//デフォルトアクション(複数指定可能)
let defaultAction = UIAlertAction(title: "デフォルト", style: UIAlertActionStyle.Default, handler: {
(action:UIAlertAction!) -> Void in
print("デフォルト")
})
//デフォルトアクション(複数指定可能)
let default2Action = UIAlertAction(title: "デフォルト2", style: UIAlertActionStyle.Default, handler: {
(action:UIAlertAction!) -> Void in
print("デフォルト2")
})
//破壊的アクション(複数指定可能)
let destructiveAction = UIAlertAction(title: "破壊", style: UIAlertActionStyle.Destructive, handler: {
(action:UIAlertAction!) -> Void in
print("破壊")
})
let destructive2Action = UIAlertAction(title: "破壊2", style: UIAlertActionStyle.Destructive, handler: {
(action:UIAlertAction!) -> Void in
print("破壊2")
})
alert.addAction(cancelAction)
// alert.addAction(cancel2Action) //これを追加すると「UIAlertController can only have one action with a style of UIAlertActionStyleCancelが発生する。
alert.addAction(defaultAction)
alert.addAction(default2Action)
alert.addAction(destructive2Action)
alert.addAction(destructiveAction)
presentViewController(alert, animated: true, completion: nil)
iPadでAction Sheetを表示する
iPadでAction Sheetを表示する場合追加のコードが必要となります。
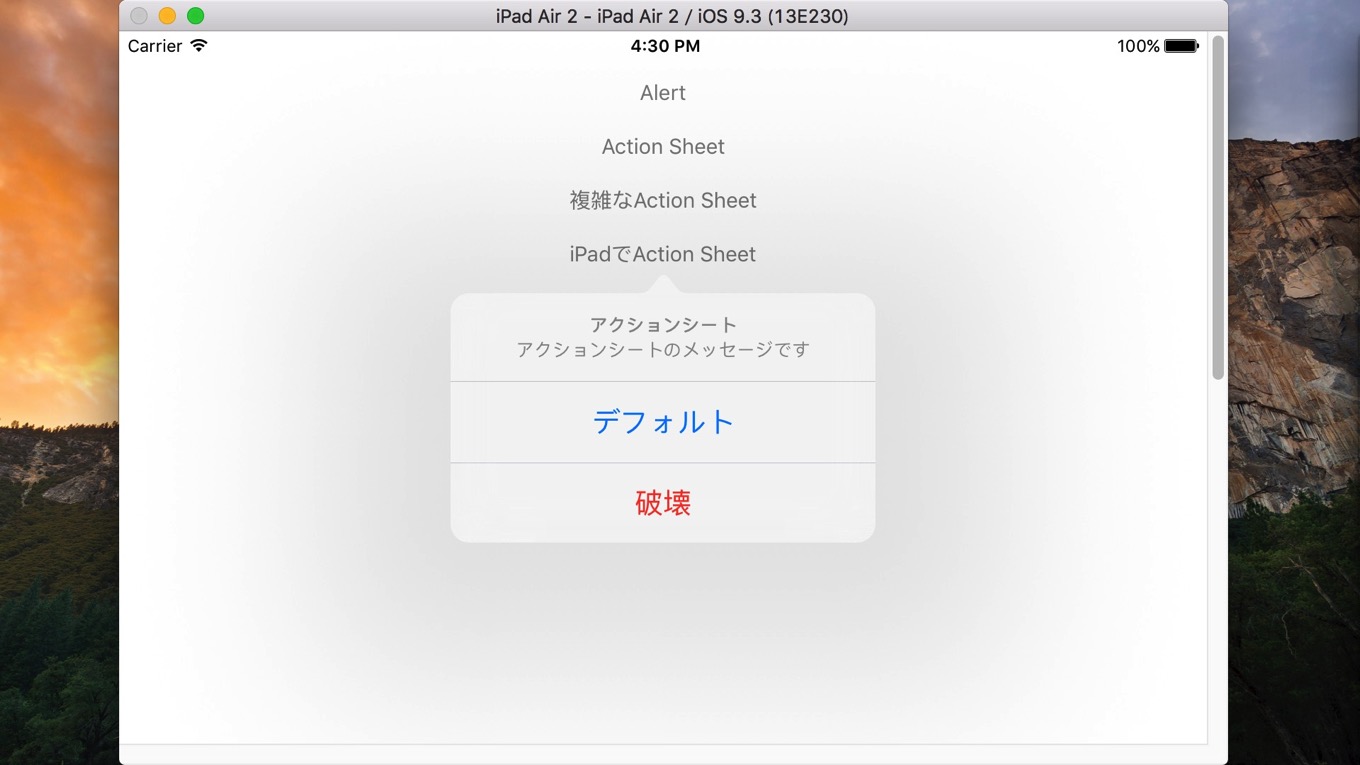
UIAlertControllerからpopoverPresentationControllerを取得しsourceViewとsourceRectに適切に値を設定します。この設定を行わないとクラッシュします。
見た目の形状が吹き出し式に変わっていることにも注目です。
@IBAction func ipadActionSheetTapped(sender: AnyObject) {
print("iPad用ActionSheetの表示")
let alert = UIAlertController(title: "アクションシート", message: "アクションシートのメッセージです", preferredStyle: UIAlertControllerStyle.ActionSheet)
if let popoverPresentationController = alert.popoverPresentationController {
//iPadの場合sourceViewとsourceRectを設定する必要あり
print("popoverPresentationController is not nil")
popoverPresentationController.sourceView = self.view
popoverPresentationController.sourceRect = sender.frame
} else {
//iPhoneの場合はnil
print("popoverPresentationController is nil")
}
//キャンセルアクション(1つだけ指定可能)
let cancelAction = UIAlertAction(title: "キャンセル", style: UIAlertActionStyle.Cancel, handler: {
(action:UIAlertAction!) -> Void in
print("キャンセル")
})
//デフォルトアクション(複数指定可能)
let defaultAction = UIAlertAction(title: "デフォルト", style: UIAlertActionStyle.Default, handler: {
(action:UIAlertAction!) -> Void in
print("デフォルト")
})
//破壊的アクション(複数指定可能)
let destructiveAction = UIAlertAction(title: "破壊", style: UIAlertActionStyle.Destructive, handler: {
(action:UIAlertAction!) -> Void in
print("破壊")
})
alert.addAction(cancelAction)
alert.addAction(defaultAction)
alert.addAction(destructiveAction)
presentViewController(alert, animated: true, completion: nil)
}